Introduction
For my small horror game I needed to save some data for the secrets in the game to work properly. I like to manage save data the same way that some old games do, as I find it’s and incredibly simple but effective way to do it.
So for Escape Floor Zero I created a SaveGame object in Unreal and there I created a single variable: A Map of Names to Integers.
In this article you will learn how to build a super simple Save System in Blueprint like this to be able to save your game progress.
Creating a SaveGame Object in Unreal Engine
To create a Save Game Object in Unreal, right click on the content browser and then choose the Blueprint category. Once there, search for SaveGame and click it.
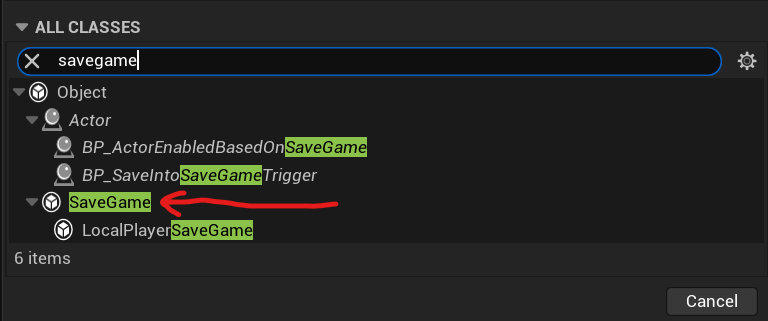
This will create a new SaveGame object in your content browser, you can name it whatever you like. All your game save data will live here.
What we will do now, is create a new variable, you can do that by pressing the "+" button on the right of the Variables section.
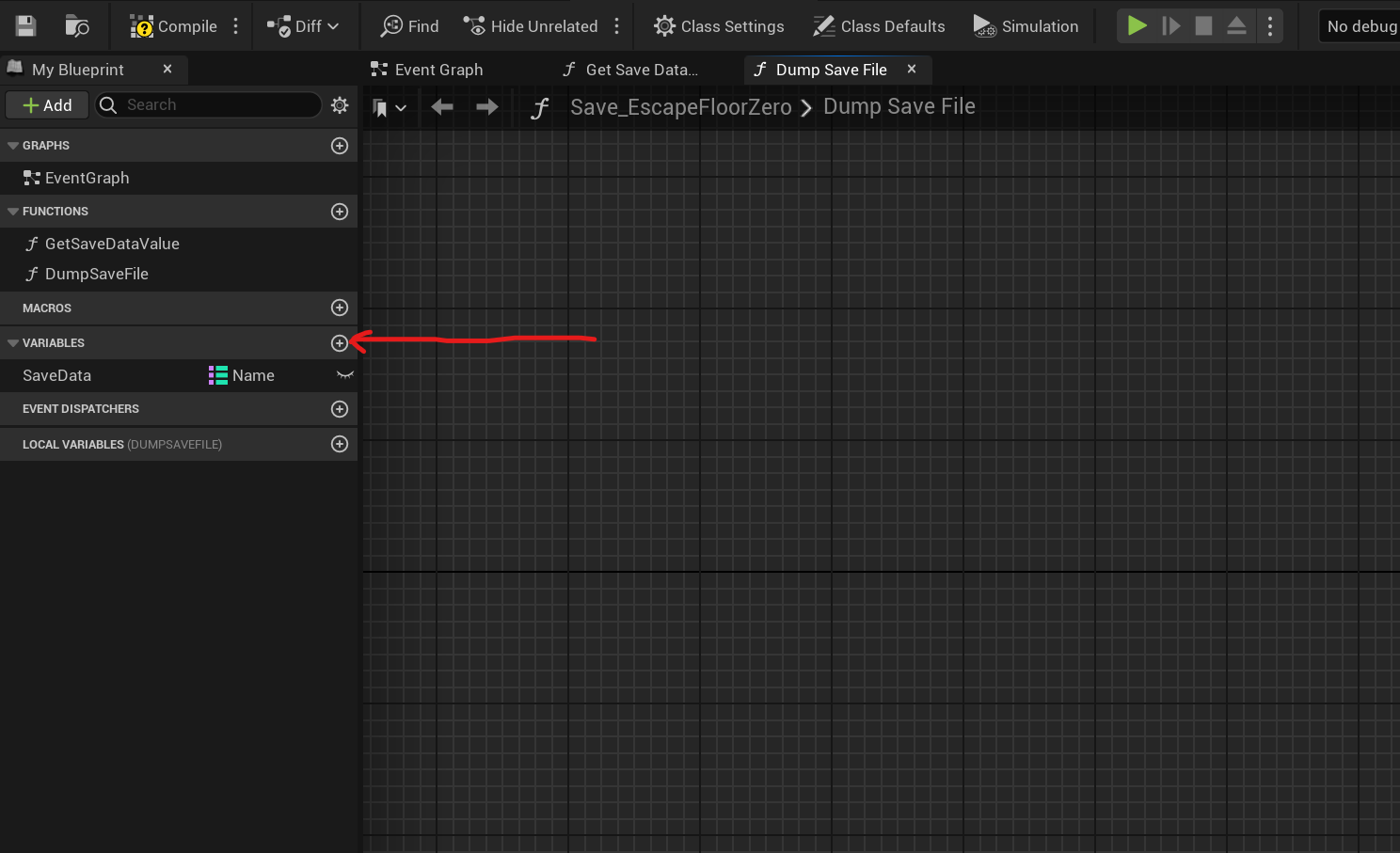
You can call this variable what you want, but as we want to store everything in here, we will call it SaveData. This variable will be a Map of Names to Integers.

And with this our Save Object is ready on the data part.
Creating the simple Save System using the Unreal SaveGame Object
Our SaveGame class is ready, now we need to use it.
The first thing we need to do is to create it or load it once the game starts. This can be done in many places ( Game Instance, Game Mode, Custom Logic... ). In this case we will do it in the Begin Play function of our Game Mode.
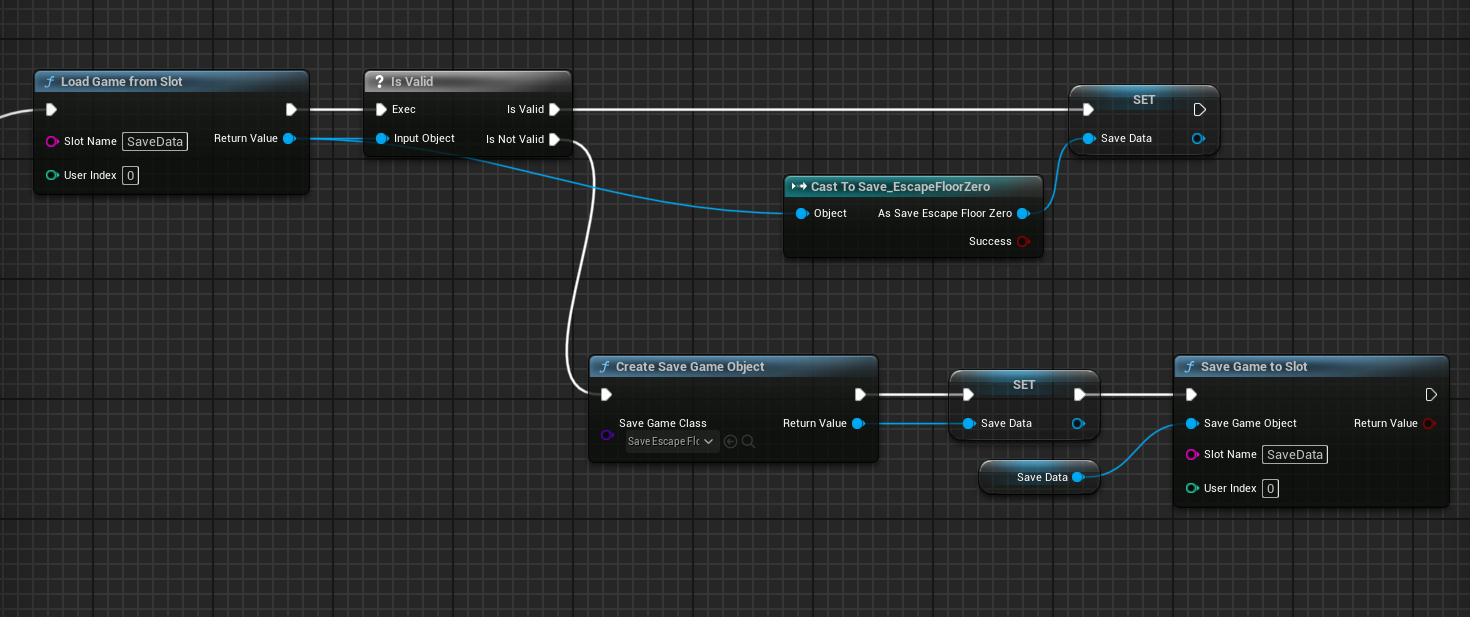
Let's break a little bit down what we are doing here:
- First we are trying to load a SaveGame file called "SaveData" ( this has nothing to do with the variable of the same name, this is the name of the actual file that will exist in the disk )
- If the file is found, the SaveGame is loaded, so we Cast it to our SaveGame type that we cerated and assign it to a variable, to be used later
- If the file is not found, this means that no SaveData exist, in this case we create a SaveGame of the type we want, store it in a variable, and write it back into disk
With these simple steps we have our save system already working, that will create a new save if no data is found or will load the data into memory if we found it in disk.
How to Read and Write data to the Save Game Object
Now that the system is ready, let's see how we can read and write data to it.
The way that we have setup things, we have a SaveGame Object that has a single variable called "SaveData" in which we will store everything, so the way this will work is the following.
If we want to store anything we will assign a "Name" to the thing we want to store and then hold its value, let's see some examples:
- Player.NumDeaths -> 3: This will store that the player has died 3 times
- VisitedLocations.SuperCoolCity -> 1: This will store that the location "SuperCoolCity" has been visited
- NPC.TownVendor.ShopLevel -> 5: The shop level for the town shop npc is at level 5
- World.DefeatedEnemies.Dungeon07.DragonMonster07 -> 1: A specific monster at a specific location is dead
We could go on, but as you can see a simple system like that is incredibly versatile in all the information it can hold.
Let's see now how to write data into the savegame.
You remember that we stored a variable to the SaveGame object in the Game Mode, now it's time to use it, to make things easier we can create some functions in the SaveGame object, it's a Blueprint Object after all.

In the end, internally we are doing this:
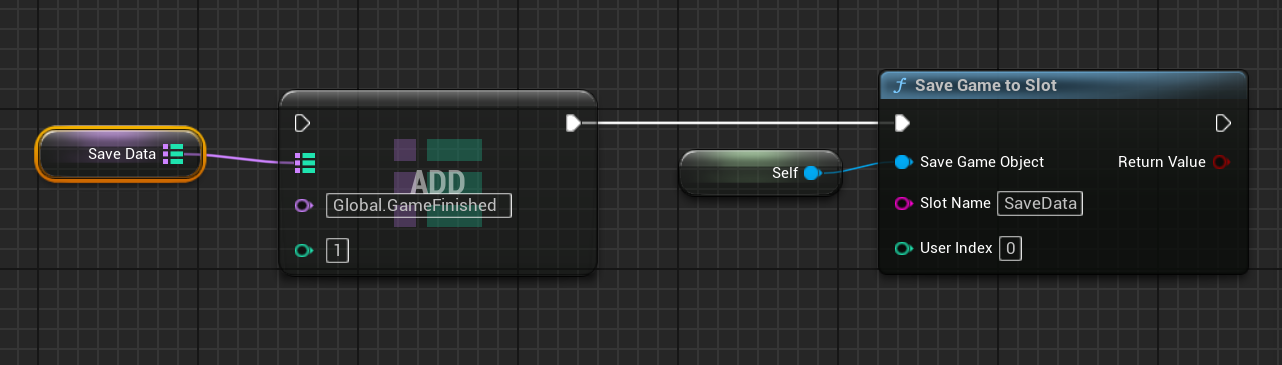
We are inserting the value in the map and then we are writing the file to disk.
You will notice that in the function I created, I added a "PerformSave?" input. This is because writing a file to disk can be expensive, so instead of always writing to disk, we can keep those changes in memory, and only write to disk in certain cases, like after some time, or if you defeat a boss, or other important events.
Reading SaveGame data is even easier, as it's simply finding it in the map.

The boolean output will tell us if the value even exists and the integer output will be the value itself.
As you can see it's incredibly easy to use and at this point the Save System is complete.
Conclusion
In this article you have learned how to implement a simple save system that is versatile and can be used for a ton of different use cases.
For you to be aware lot's of games use systems similar to this, if you are familiar with Pokemon or RPG Maker you will have already noticed that this system is really similar to what they use there.
That being said other modern games use systems similar to this, although a little bit more complex that is how Zelda: Breath of the Wild stores the enemies you have defeated, locations visited or it's Quest and World state.

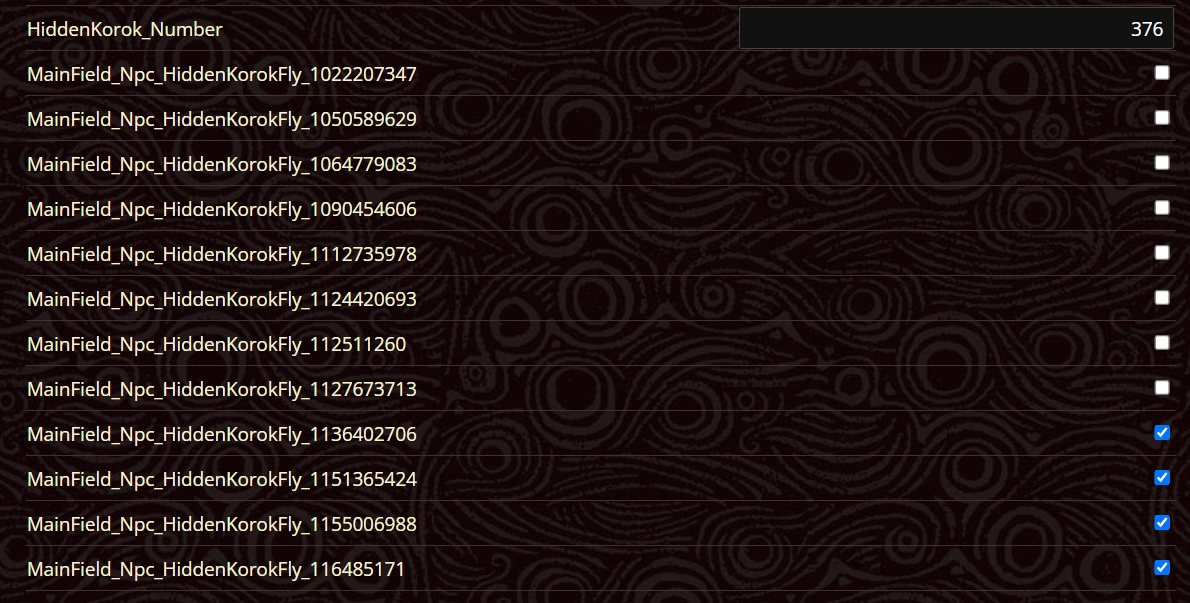
For more complex use cases you may need something else entirely than a system built in 10 minutes, but for a simple game it can be a powerful way to manage your save data.
I hope this article has been helpful to you. See you next time!